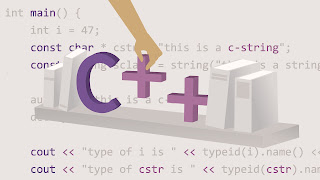
The containers I shall be covering include:
CONTAINERS
- String Streams
- Map
- Multiset
- Priority Queue
String Streams
Stringstream is the processing of input/output strings. The stringstream class in C++ allows a string object to be treated as a stream. It is used to operate on strings.By treating the strings as streams we can perform extraction and insertion operation from/to string just like cin and cout streams.
C++ provides two interesting objects for it: ‘istringstream’ (input stringstream ) and ‘ostringstream’ (output stringstream). Both of these are declared in #include< stream >.
Insertion And Extraction Operations Using stringstream
Insertion
(i) Using Insertion Operator (<<)
Data is assigned to stringstream buffer using << operator.
(ii)Using str(string) Function
Str function can also be used for assigning data to the stringstream buffer. The str function takes the data string as an argument and assigns this data to the stringstream object.
Extraction
(i) Using Extraction Operator (>>)
Stringsream data can be displayed using >> operator.
(ii)Using str() Function
We can use the str() function to get the data out of stringstream.
We can use the str() function to get the data out of stringstream.
Demonstration of Insertion and Extraction Operators :
Elements of map and set are always sorted in ascending by default.
Traversing map is easy with iterator, here iterator will be a pair of key and value. So, to get the value use ( it->first ) or ( it->second ).
Click Here Run and Check The Output Of Above Code
Map
Map is quite similar to set, except it contains not just values but pairsv<key, value>. Map ensures that at most one pair with specific key exists. Therefore, a map contains keys in a specific order and all the keys are unique with some value.Internally map and set are almost always stored as red-black trees.Elements of map and set are always sorted in ascending by default.
Traversing map is easy with iterator, here iterator will be a pair of key and value. So, to get the value use ( it->first ) or ( it->second ).
Some Built-In Functions
- begin(): Returns an iterator to the first element in the map.
- size(): Returns the number of elements in the map.
- empty(): Returns a boolean value indicating whether the map is empty.
- insert( pair(key, value)): Adds a new key-value pair to the map. An alternate way to insert values in the map is:map_name[key] = value;
- find(val): Gives the iterator to the element val, if it is found otherwise it returns m.end()
- erase(iterator position): Removes the element at the position pointed by the iterator.
- erase(const g): Removes the key value g from the map.
- clear(): Removes all the elements from the map.
Implementation :
Click Here To Run the above code and check it's output
Multiset
Multisets are containers that store elements following a specific order, and where multiple elements can have equivalent values or there can be duplicate elements in it.
Implementation :
Click Here To Run & Check Output
Priority Queue
Priority queues are a type of container adaptors, specifically designed such that its first element is always the greatest/smallest of the elements it contains, according to some strict weak ordering criterion.
Priority Queue is used mostly in questions where the order of elements is either strictly increasing or decreasing. Insertions take place in a priority queue using push() function and deletion take place using pop().
Greatest/Smallest value element is always present on the top of the priority queue and pop() also deletes the element present on the top of priority queue.
Implementation
Click Here To Run & Check Output
This is not the end of STL but the beginning... stay tuned for more stuff to come.
Happy Coding..!!
This is not the end of STL but the beginning... stay tuned for more stuff to come.
Happy Coding..!!
Comments
Post a Comment
Your Feedback Will Help Us To Improve...